Final Fixes and Buoyant Boats
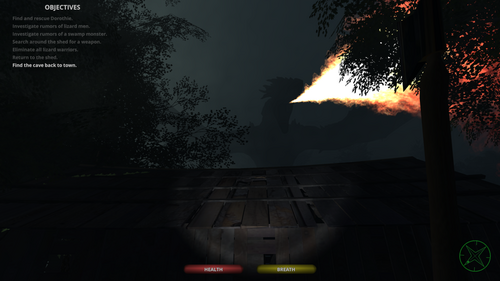
So, I'm done. There are some issues with the lighting that I can't sort out with Aura 2. It looked fine before, but I've made some tweak somewhere that now ends up producing blocky, chunky shadows when one faces the sun. I still haven't sorted out the underwater fog issue. This seems to be a known issue with Aquas, but unfortunately, the developer has gone AWOL. Too bad, because it's a really nice tool otherwise.
I slowed the opening boat ride down a bit and added buoyancy physics to be boat, because why not. The buoyancy physics script works better than I had hoped. It is a hack job, loosely based on real physics, but it looks believable which is really what we're after with most game physics, right? I've included the script below.
Regardless, I'm done with this prototype. It was fun to create, and it helped me work on a variety of skills. I'll have a formal postmortem posted later next week. As always, I appreciate feedback and comments.
/******************************************************************************
* BuoyancyPhysics applies an upward buoyant force to an object based on the
* level of a user-supplied water plane. The buoyant object MUST have a
* rigidbody component attached to it.
*
* J. Douglas Patterson, 26-MAR-2020
* http://www.redhandgames.com/
*
* ***************************************************************************/
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
[RequireComponent(typeof(Rigidbody))]
public class BuoyancyPhysics : MonoBehaviour
{
/* These variables are exposed in the Inspector so that the designer *
* can adjust the values to best suit the buoyant game object. */
public GameObject waterPlane; //Game object representing the water in which you wish to float your boat
public float beam = 1.0f; //Width of the boat (m)
public float length = 2.0f; //Length of the boat (m)
public float waterDensity = 1.0f; //Specific density of the fluid
public float gravity = 9.8f; //Acceleration due to gravity (m/s^2)
public float torsion = 0.1f; //Restoring coefficient for the buoyant torque
Rigidbody rb_Boat; //Rigidbody component for the boat
//Variables for calculating and applying the upward buoyant force
float boatArea; //Topside area of the boat (m^2)
float boatDisplacement; //Volume of water displaced by the boat (m^3)
Vector3 buoyantForce; //Upward buoyant force on the boat (N)
//Variables for calculating and applying the restoring torque for self-righting
float dRotX; //Pitch angular displacement of the boat (degrees)
float dRotZ; //Roll angular displacement of the boat (degrees)
Vector3 restoringTorque; //Torque applied to restore the boat to it's upright position
// Start is called before the first frame update
void Start()
{
//Store the rigidbody component for the force.
rb_Boat = GetComponent<Rigidbody>();
//Initialize the geometry and initial submerged volume for the boat.
boatArea = beam * length;
boatDisplacement = boatArea * (waterPlane.transform.position.y - transform.position.y);
if (boatDisplacement < 0.0f) boatDisplacement = 0.0f;
//Calculate the inital buoyant force.
buoyantForce = new Vector3(0.0f,boatDisplacement * waterDensity * gravity,0.0f);
}
// Update is called once per frame
void FixedUpdate()
{
//Calculate the displaced water volume
boatDisplacement = boatArea * (waterPlane.transform.position.y - transform.position.y);
if (boatDisplacement < 0.0f) boatDisplacement = 0.0f;
//Calculate and apply the buoyant force
buoyantForce = new Vector3(0.0f, boatDisplacement * waterDensity * gravity, 0.0f);
rb_Boat.AddForce(buoyantForce,ForceMode.Force);
//Calculate pitch angular displacement from equalibrium
if (transform.localEulerAngles.x < 180.0f)
{
dRotX = transform.localEulerAngles.x;
}
else
{
dRotX = transform.localEulerAngles.x - 360.0f; //Use the short angle back to equalibrium
}
//Calculate roll angular displacement from equalibrium
if (transform.localEulerAngles.z < 180.0f)
{
dRotZ = transform.localEulerAngles.z;
}
else
{
dRotZ = transform.localEulerAngles.z - 360.0f; //Use the short angle back to equalibrium
}
//Calculate and apply the restoring torque
restoringTorque = new Vector3(-1f * torsion * beam * dRotX, 0.0f, -1f * torsion * length * dRotZ);
rb_Boat.AddTorque(restoringTorque, ForceMode.Force);
}
}
Files
Get Bayou Kaiju
Bayou Kaiju
Run through the bayou, fight lizard men, and rescue your friend's daughter from the giant swamp monster.
Status | Prototype |
Author | J. Douglas Patterson |
Genre | Action, Survival |
Tags | Action-Adventure, bayou, kaiju, suspense, swamp |
More posts
- Bayou Kaiju PostmortemApr 14, 2020
- Final FINAL Fixes ...for nowApr 04, 2020
- Smoke on the WaterMar 25, 2020
Leave a comment
Log in with itch.io to leave a comment.